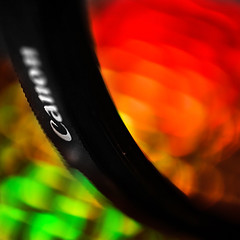
After you learn how to get data out of a table with the SELECT command, you’ll soon ask the question, how do I limit the number of results I get back. Well, If you don’t ask the question, your DBA will. I don’t think he or she would like it if you only ever used SELECT without learning the WHERE clause. Adding this clause to your query will let you limit the number of rows the server will return. You’ll be able to identify certain characteristics the results will have in common.
Let’s go back to our copy of AdventureWorks. This time, we’re going to look at contacts stored in our database. We’re going to use the Person.Contact table. We’re going to look for contacts with the last name Lowder.
SELECT FirstName, LastName FROM Person.Contact WHERE LastName = 'Lowder'
output
FirstName LastName --------- -------- Shannon Lowder
The WHERE clause will let you limit the number of rows based on any column in the table you’re querying. Rather than showing every first and last name in the table, this query only returns the first and last names for contacts who have the last name lowder. Currently in my database, I only have one record. My record. If there had been any other Lowder in this table, say my parents, or sister, those records would have been returned by this query.
For now, you’ll only be able to limit your results based on the values in the columns. You have many comparison operators you can use. In my above example you saw the equals operator. Let’s show you a few more
Comparison Operators
- =
- >, >=
- <, <=
- <>, !=
- LIKE
- IN (…)
- BETWEEN
The first four should look familiar if you’ve been through a few math classes, but the last three are specific to SQL. Let’s walk through how to use these.
LIKE
LIKE allows you to do partial matching. There are going to be times where you know only part of the value you’re searching for. Let’s say you know you have a contact whose last name starts with a “s”, but you can’t remember the rest. You can do that look up by using the LIKE operator and a wild card.
SELECT FirstName, LastName FROM Person.Contact WHERE LastName LIKE 's%'
output
FirstName LastName --------- -------- Margaret Smith Deanna Sabella Lane Sacksteder Peter Saddow

There are other records, but I stopped after the 4th, you get the idea. If not… Run this query for yourself.
I mentioned a wild card. If you’ve worked in DOS, you may remember DIR *.txt. The * is the wild card in dos. In Microsoft SQL, the wild card is %. We wanted to see everything where the last name started with “s” and had anything after that.
The LIKE operator can do some pretty advanced things. If you are familiar with regular expressions, you should know that the LIKE comparator works with regular expressions. I really need to cover this in a blog post.
IN
Let’s say you had a list of records you were looking for. For example, you want to find people with a last name of Smith, Jones, or Adams. Pretty common names, right? If you wanted to do this kind of look up you will want to use the IN (…) comparator, you can then list the values you want returned.
SELECT FirstName, LastName FROM Person.Contact WHERE LastName IN ('Smith','Jones','Adams')
output
FirstName LastName --------- -------- Frances Adams Margaret Smith Carla Adams Jay Adams Robert Jones
I’ve truncated the results here. But feel free to run this query yourself to see the full results.
When you use the IN clause, you’re not going to have the same flexibility you have with LIKE. So don’t be too disappointed when you switch from LIKE to IN. They both have their uses. Learning when yo use each… that’s the key.
BETWEEN
When you want to search for items that occur in a range of values, you’ll use BETWEEN. I use this a lot when doing date based searches. Such as who was paid BETWEEN ‘1/1/2005′ AND ’12/31/2005’. I also use it for value ranges like, show me all the products that have a list price between $2,500 and $5,000.
SELECT Name, ListPrice FROM Production.Product WHERE ListPrice BETWEEN 2500 and 5000
output
Name ListPrice ---------------- --------- Road-150 Red, 62 3578.27 Road-150 Red, 44 3578.27 Road-150 Red, 48 3578.27
Play around with the WHERE clause a bit. If you have any questions, please, feel free to comment below! I’m here to help you grow stronger in the ways of The Force, err… SQL.
Previous: SELECT | Next: SELECT, Filtering Results (Part 2) |